How to Import Class from Another Python File
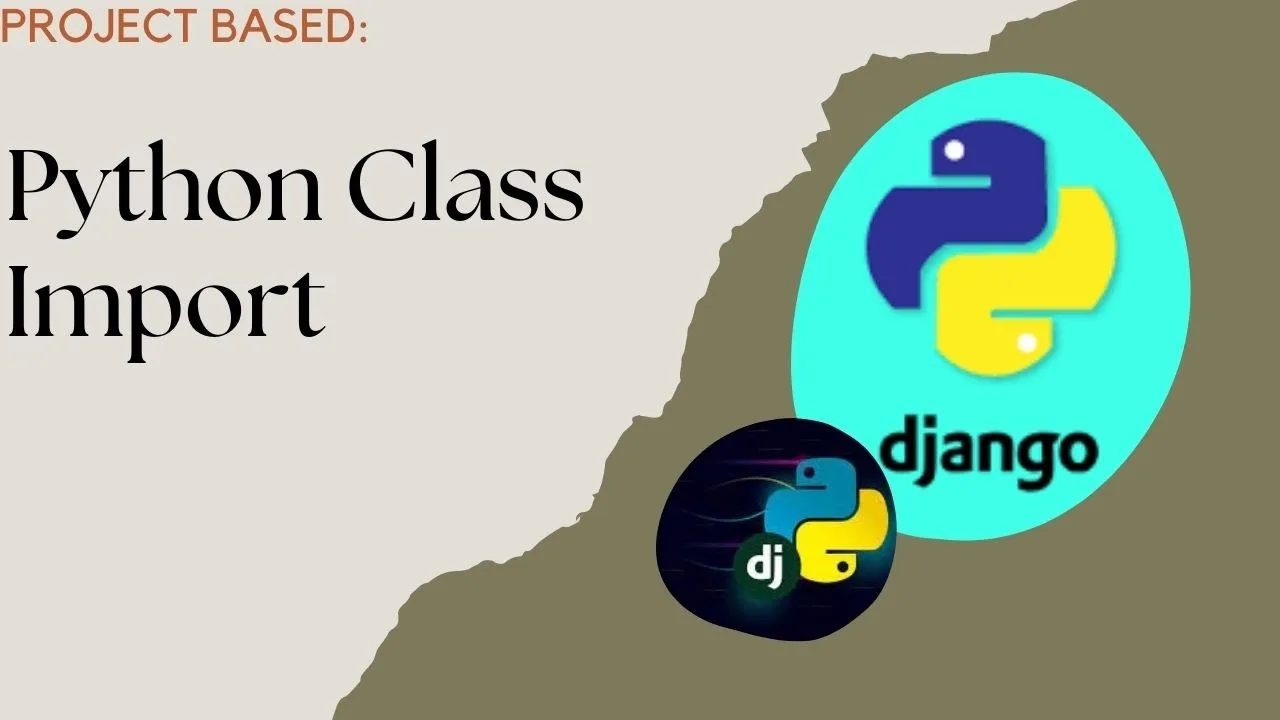
Assuming you have a file named “example.py” with a class called “Example”:
- Go to the directory where the file you want to import is located
- Type “import” followed by the name of the file you want to import
- Press Enter
Unable to Import Class from Another File Python
If you’re working with Python, you may find yourself unable to import a class from another file. This can be frustrating, but there are a few things you can do to troubleshoot the issue.
First, make sure that the file you’re trying to import is in the same directory as the script you’re running.
If it’s not, you’ll need to specify the full path to the file.
Next, check that the file you’re importing contains the class you’re trying to use. It’s possible that the class has been renamed or moved to a different location in the file.
Finally, if all else fails, try restarting your Python interpreter. Sometimes this can clear up issues with imports.
If you’re still having trouble importing a class from another file, Python’s documentation may have more information on how to troubleshoot your specific issue.
Python Import Class from File in Another Directory
If you want to import a class from a file in another directory, you need to add the directory that contains the file to your Python path. You can do this by adding the following line to your Python script:
import sys
sys.path.append(‘/path/to/directory’)
Once you have added the directory to your Python path, you can import the class from the file using the following syntax:
Python Import Class from Another File in Same Directory
Python is a programming language that allows for easy manipulation of data. One of its key features is the ability to import classes from other files in the same directory. This can be extremely useful when working with large projects that have many different modules.
To import a class from another file in the same directory, we first need to use the “import” keyword. This tells Python that we want to use a class from another file. Next, we specify the name of the file that we want to import the class from.
Finally, we use the “class” keyword followed by the name of the class that we want to import.
For example, let’s say we have a file called “main.py” and another file called “util.py” in the same directory. We can import the “MyClass” class from “util.py” into “main.py” like this:
import util MyClass = util .
How to Import Class from Another Python File in Jupyter Notebook
If you’ve ever wanted to import a class from another Python file in Jupyter Notebook, you know it can be a bit tricky. In this post, we’ll show you how to do it with just a few simple steps.
First, open your Jupyter Notebook and create a new notebook.
Then, open the file that contains the class you want to import. Copy the code for the class into your clipboard.
Next, open your new notebook and create a cell.
In the cell, type “import” followed by the name of the class you’re importing. For example, if we’re importing the “MyClass” class from “myfile.py”, we would type:
import myfile.MyClass
Finally, press Shift+Enter to run the cell and import the class into your notebook!
Python Import Class from Another File No Module Named
If you’re just getting started with Python, you may not know how to import a class from another file. It’s actually pretty simple, but there are a few things to keep in mind.
First, make sure that the file you want to import is in the same directory as your Python script.
If it’s not, you’ll need to specify the full path to the file.
Second, when you import a class from another file, you need to use the syntax “from
Finally, if you try to import a class that doesn’t exist in the specified file, you’ll get an error saying “No module named
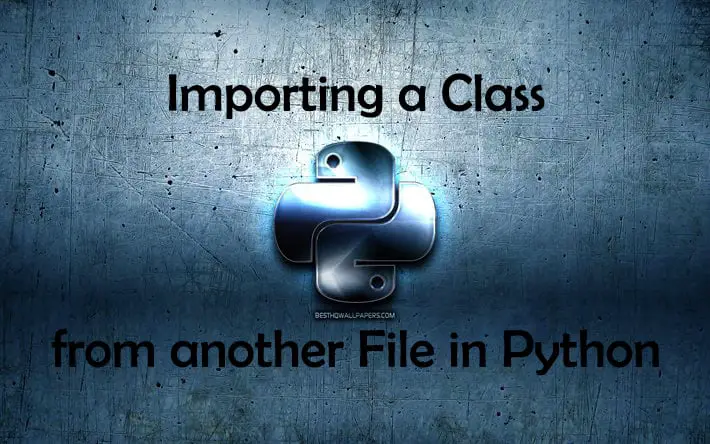
Credit: coderslegacy.com
Can You Import Classes from Another Python File?
Yes, you can import classes from another Python file. To do so, you first need to make sure that the file containing the class is in the same directory as your main Python file. Then, you can use the “import” keyword to import the class into your main Python file.
For example:
main.py:
import my_class
How Do You Import a Class from a Python File in Another Python File?
Assuming you have a Python file in the same directory as your current Python file, you can import it using the following code:
import filename
You can also import classes from Python files in other directories.
To do this, you must first add the directory to your system path. You can do this by adding the following line of code to your current Python file:
import sys sys.path.append(‘directory’)
After adding the directory to your system path, you can import any class from any Python file in that directory using the following code:
How to Import Class from Another Python File in Different Directory?
Assuming you have a directory structure like the following:
myproject/
__init__.py
settings.py
urls.py
myapp1/
__init__.py
views.py
models.py
utils/
__init__.py
util1.py
util2.py
myapp2/
…
How Do I Import Code from Another File in Python?
In Python, importing code from another file is relatively simple. First, we need to make sure that the file we want to import is in the same directory as our current file. If it’s not, we can specify the path to the file using one of two methods.
The first method is to use an absolute path, which will always point to the same location regardless of where our current file is located. The second method is to use a relative path, which will be interpreted based on our current file’s location.
Once we have our imports sorted out, we can use the “import” keyword followed by the name of the module or package we want to import.
For example, if we wanted to import the “math” module, we would write:
import math
This would give us access to all of the mathematical functions defined in that module.
We could then use them by writing something like “math.sqrt(4)” which would calculate and return the square root of 4 (which is 2).
If there are specific functions or variables within a module that we want to import, we can list them after the “import” keyword like so:
from math import sqrt, pi
This would give us access just to those two functions from the math module (and any other modules they happen upon). We could then call them directly without having to prefix them with “math.”, like so:
Python Class Import Class import classes to another file in Python|| Must Watch – English
Conclusion
If you need to use a class that’s defined in another file, you can import it into your current file. To do this, you first need to add an import statement at the top of your file. The import statement looks like this: from module_name import class_name.
module_name is the name of the file (without the .py extension) and class_name is the name of the class you want to use.