How to Implement Multiple Interfaces in Java

There are two ways to implement multiple interfaces in Java: by using separate interface declarations or by using a single, combined interface declaration.
When using separate interface declarations, each interface must be implemented as a separate class. For example, if you have two interfaces, Interface1 and Interface2, you would need to create two classes that implement each interface:
class Implementation1 implements Interface1 { … } class Implementation2 implements Interface2 { … }
You can then use either Implementation1 or Implementation2 wherever an instance of Interface1 or Interface2 is required.
If you only want to create one implementation class, you can use a single, combined interface declaration.
- Assuming you have two interfaces, Interface1 and Interface2, that you want to implement in your Java class MyClass: 1) Declare that MyClass implements both Interface1 and Interface2
- For example: public class MyClass implements Interface1, Interface2 { // Class body goes here } 2) Implement all methods required by both interfaces
- In the case of default methods, you can choose which one to override
- 3) If an interface requires a static method to be implemented, create a static method with the same signature in your class
Multiple Inheritance Using Interface in Java
Inheritance is a mechanism in java by which one class can acquire the properties (methods and fields) of another. There are various types of inheritance in java but multiple inheritance using interface is not supported by java. Though multiple inheritance is supported by many other programming languages, it was dropped from java due to the ambiguity that it might cause.
In this blog post, we will understand what multiple inheritance is and whyjava does not support it.
Multiple Inheritance: It is a feature of some object-oriented programming languages in which an object or class can inherit characteristics and behavior from more than one parent object or class.
Why Java does not support Multiple Inheritance?
The main reason for dropping multiple inheritance from java was to reduce the complexity and ambiguity that it might cause. Let us understand this with an example. Consider a scenario where there are two classes A and B with methods m1() and m2().
Now, consider another class C which inherits both these classes i.e., Class C extends Class A & Class B. Now if we invoke method m1() from class C, there will be ambiguity as to whether method m1() of Class A or method m1() of Class B should be invoked? To avoid such scenarios,multiple inheritance using interface was dropped from java .
However, multiple inheritance can be achieved in java using interfaces .
An interface in java is like a blueprint of a class which has static constants and abstract methods only. Since aninterface cannot have any implementation, they are often referred to as pure abstraction . So when we say that multiple inheritance can be achieved using interfaces , what we mean is that aclass can implement more than one interfaces .
Two Classes Implementing Same Interface Java
When two classes implement the same interface in Java, they are said to be “functionally equivalent”. This means that the two classes can be used interchangeably, without any loss of functionality.
There are some important things to keep in mind when using functionally equivalent classes:
1. The interfaces must be identical. This means that all methods and fields must have the same signature (name, return type, etc).
2. The behavior of the methods must be identical.
This includes side effects (such as modifying fields) and return values.
3. The state of the objects must be identical. This means that all fields must have the same values after a method is invoked.
4. The timing of events must be identical. This means that methods must be invoked in the same order and at the same time intervals.
How to Implement Interface in Java
An interface is like a template that you can use to create a Java class. It contains a set of methods that your class must implement. By using an interface, you can create a relationship between two classes that wouldn’t otherwise be possible.
To implement an interface in Java, you use the keyword “implements.” This is followed by the name of the interface you want to implement. For example, if you wanted to implement the Interface1 interface, your code would look like this:
public class MyClass implements Interface1 {
//Your code goes here
Can a Class Implement Multiple Interfaces in C#
NET
In the world of programming, an interface is like a contract. A class can implement multiple interfaces, just as it can inherit from multiple classes.
This allows for greater flexibility in how that class can be used.
For example, let’s say you have a class called Car. This class might implement the following interfaces:
– ITransport: Car can be used to transport people or goods from one place to another.
– IDriveable: Car can be driven by a person.
– IComputable: Car has certain computable properties, such as its speed or fuel efficiency.
Each of these interfaces represents a different set of functionality that the Car class provides. Implementing multiple interfaces is a way of saying “this class can do more than one thing”.
Variables in Interface in Java
In Java, a variable in an interface is by default public, static and final. This means that when you create a variable in an interface, you cannot change its value. It is also accessible to all classes that implement the interface.
A static variable is a variable that is associated with a class, not with an instance of the class. A static variable can be accessed without creating an instance of the class.
A final variable is a constant.
Once it is assigned a value, it cannot be changed.
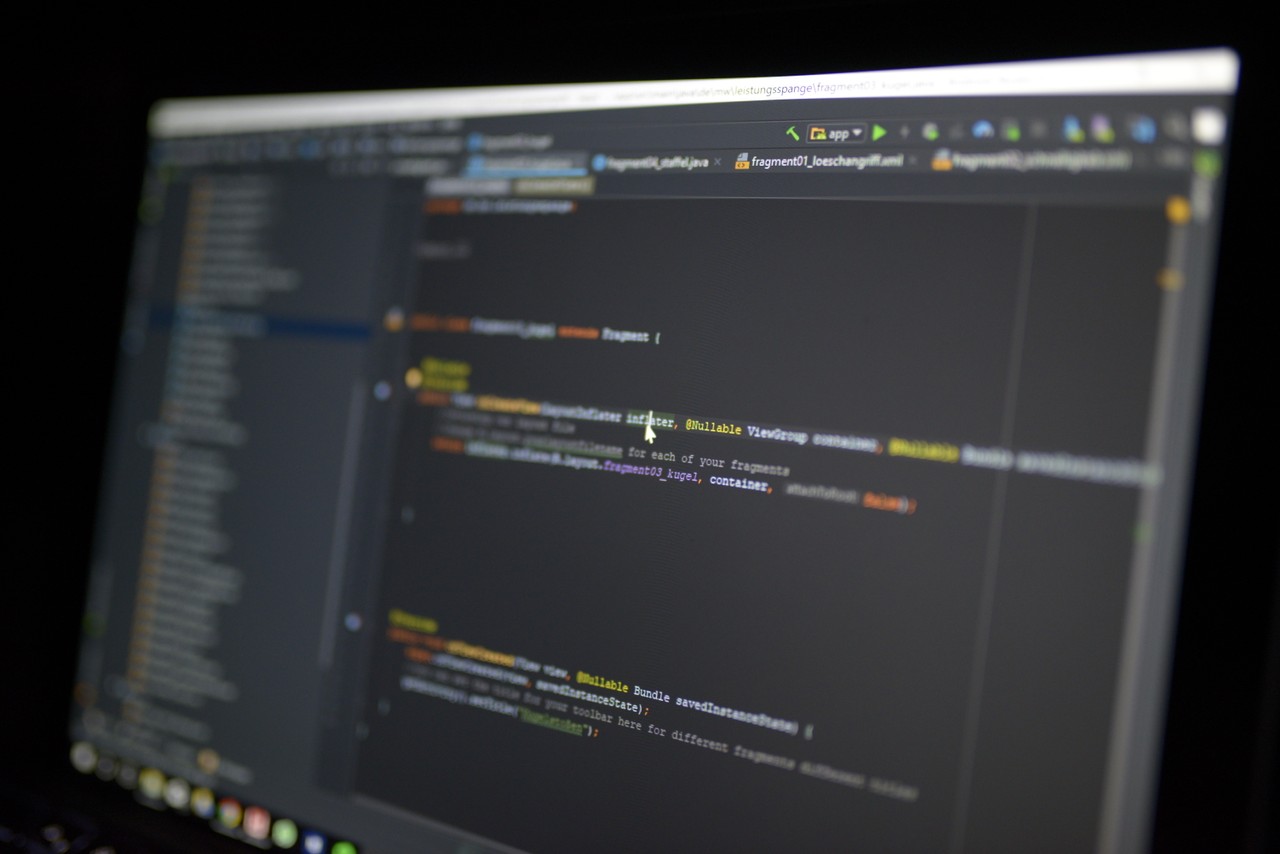
Credit: www.freecodecamp.org
Can I Implement Multiple Interfaces in Java?
Yes, you can implement multiple interfaces in Java. This is called “multiple inheritance.” However, there are some restrictions on how you can do this.
First, you can only inherit from one class and one interface at a time. So if you have a class that implements multiple interfaces, each interface can only have one parent class. Second, all of the interfaces must be compatible with each other.
That is, they must all use the same type of object for their parameters and return values. Third, if any of the interfaces contain methods with the same name and signature, then those methods must have the same behavior in all of the classes that implement them.
How Many Interfaces Can a Class Implement in Java?
A class in Java can implement any number of interfaces. There is no limit to the number of interfaces a class can implement. This is because a class can only inherit from one superclass, but it can implement multiple interfaces.
Can We Use Multiple Interfaces in One Class?
Yes, we can use multiple interfaces in one class. By doing so, we are effectively creating what is known as a “multiple inheritance” scenario.
While this can be powerful and offer some interesting possibilities, it also comes with its own set of potential problems.
Most notably, the “diamond problem” can occur when two superclasses (in our case, interfaces) have conflicting method implementations. In such a situation, it is ambiguous as to which implementation should take precedence.
Therefore, while multiple interface inheritance is possible in Java, it is generally not considered to be best practice and should be avoided if possible.
Can We Implement Multiple Interfaces in Java 8?
Yes, we can implement multiple interfaces in Java 8. Prior to Java 8, a class could only implement a single interface. However, starting with Java 8, a class can implement multiple interfaces.
There are two ways to achieve this:
– The first way is to use the “implements” keyword for each interface that you want the class to implement.
– The second way is to use the “extends” keyword for the first interface, and then use the “implements” keyword for each subsequent interface.
Here’s an example using the first method:
public class MyClass implements Interface1, Interface2 {
// body of class goes here
}
And here’s an example using the second method:
public class MyClass extends Class1 implements Interface2 {
Java for Testers – Part 208 – Implementing Multiple Interfaces
Conclusion
In Java, it is possible to implement multiple interfaces. This can be done by separating the interface names with a comma. For example, if you wanted to implement the Serializable and Cloneable interfaces, you would do so like this:
public class MyClass implements Serializable, Cloneable {
//…