How to Handle Stale Element Exception

The stale element exception is a common error when working with webdriver. It occurs when the element you are trying to interact with is no longer in the DOM. There are a few ways to handle this exception:
1) Find the element again using a different method. For example, if you originally found the element by its ID, try finding it by XPath or CSS selector instead.
2) Use the WebDriverWait class to wait for the element to be visible on the page before interacting with it.
3) If none of the above methods work, try refreshing the page and then finding the element again.
- Stale element exception is a type of error that occurs when an element on the page is no longer valid or does not exist
- This can happen if the element has been deleted, refreshed, or the page has been loaded again
- To handle this exception, you can try to find the element again or wait for it to be available before performing any actions on it
How to Fix Stale Element Reference Exception in Selenium Webdriver Java
If you’ve ever gotten a StaleElementReferenceException while using Selenium Webdriver, you know how frustrating it can be. This error can be caused by a number of things, but fortunately there are a few ways to fix it.
One way to fix a StaleElementReferenceException is to simply find the element again.
This can be done by using the findElement() method on the WebDriver instance. For example:
WebElement element = driver.findElement(By.id(“some-id”));
// do something with element
…
// later on…
try {
element = driver.findElement(By.id(“some-id”)); // this will throw a StaleElementReferenceException if the element has gone stale
// do something else with element
…
} catch (StaleElementReferenceException e) {
//element has gone stale, find it again and do something with it }
Alternatively, you can use the refresh() method on an Element object to try and refresh the page before attempting to interact with the element again:
Stale Element Reference: Element is Not Attached to the Page Document
A Stale Element Reference Exception is thrown in WebDriver when the element you are trying to access is no longer attached to the DOM.
This can happen for a number of reasons, but most commonly it is because the element has been removed from the page by JavaScript after the page has loaded. It can also happen if the web page you are trying to interact with has been refreshed or reloaded before WebDriver could interact with the element.
If you are getting a StaleElementReferenceException, then it means that your code is trying to interact with an element that is no longer present on the page. You will need to find another way to reach your desired element, such as by using a different locator strategy.
Stale Element Reference: Element is Not Attached to the Page Document Selenium Python
If you’ve ever seen the error message “stale element reference: element is not attached to the page document” in Selenium, then you know how frustrating it can be. This error can be caused by a number of factors, but most often it is caused by a change in the HTML structure of the page that you are trying to interact with.
There are a few ways to handle this error, but the most common is to use the WebDriver’s execute_script() method to re-attach the element to the page.
For example, suppose you have an element on a page that you want to click on:
click me
You would find this element and click on it like so:
link = driver.find_element_by_id(“some-link”)
link.click()
However, if the HTML structure of the page changes and the “some-link” element is no longer where it used to be, then you will get a stale element reference error when you try to click on it.
To workaround this, you can use execute_script() to re-attach the element to the page before clicking on it:
link = driver.find_element_by_id(“some-link”)
How Do You Fix Stale Element Reference: Element is Not Attached to the Page Document
If you’ve ever received a Stale Element Reference error while trying to automate testing with Selenium, you know how frustrating it can be. This error can occur for a number of reasons, but the most common reason is that the element you’re trying to interact with is no longer attached to the page document. In other words, the element has been “stale.”
There are a few ways to fix this issue. The first is to simply find the element again using one of Selenium’s findElement methods. This will cause Selenium to look for the element on the page again and, if it finds it, will re-attach it to the page document.
Another way to fix this issue is to use one of Selenium’s wait methods before interacting with the element. This will cause Selenium to wait until the element is attached to the page document before trying to interact with it.
Finally, you can try using JavaScriptExecutor to execute a script that interacts with the element directly.
This bypassesSelenium altogether and should work even iftheelementisn’t attachedto t hepage document .
Regardless of which method you choose , staleelementreferenceerrorsare annoyingbut hopefully now you have some idea s on howto fix them .
Stale Element Reference: Element is Not Attached to the Page Document Robot Framework
Robot Framework is a generic test automation framework for acceptance testing and acceptance test-driven development (ATDD). It has easy-to-use tabular test data syntax and it is extendable with Python.
Selenium2Library is one of the most popular Robot Framework libraries.
Selenium2Library runs tests in a real browser instance. This makes tests slower, but also more realistic. Browser instances can be controlled with desired_capabilities .
One common issue people run into when using Robot Framework Selenium2Library is “Stale Element Reference: Element is not attached to the page document”. This error occurs when an element that was previously found no longer exists in the HTML code on the page. The most likely cause of this error is that the element was deleted from the HTML code or its location on the page changed.
There are several ways to fix this issue:
1) Find the element again using another method such as xpath or css selector.
2) If you are looping through a list of elements, add a wait between each iteration to give time for the page to load completely before trying to find the next element.
3) Modify your locator strategy to be more specific so that it only finds one element on the page instead of multiple elements.
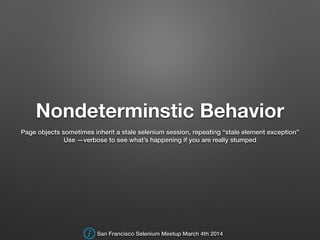
Credit: www.slideshare.net
How Do You Overcome Staleelement Exception?
If you’re a Selenium user, you’ve probably encountered the StaleElementException. This error occurs when an element in the page has been replaced by another element, and Selenium is no longer able to interact with it. In this post, we’ll take a look at how to overcome this exception and get your tests back on track.
The first step is to identify the root cause of the issue. In most cases, the StaleElementException is caused by one of two things: either the element has been replaced by another element on the page, or the page has been refreshed. Once you’ve identified the root cause, you can take steps to fix it.
If the issue is that the element has been replaced by another element, then you need to find a way to locate the new element. This can be done using Selenium’s built-in By class or by writing your own custom locator strategy. Once you’ve located the new element, you can update your test code to reference it instead of the old one.
If the issue is that the page has been refreshed, then you need to find a way to wait for all elements on the page to be reloaded before interacting with them. This can be done using Selenium’s WebDriverWait class or by writing your own custom waiter strategy. Once all elements have been loaded successfully, you can continue with your test as normal.
What is Stale Element Exception in Selenium And How to Handle It?
When working with the Selenium webdriver, you may have come across the “stale element exception”. This exception occurs when an element on a webpage is no longer attached to the DOM. In other words, if you try to interact with an element that has been deleted, moved, or is no longer visible, you will get this exception.
There are a few ways to handle the stale element exception. The first is to simply refresh the page and try again. If that doesn’t work, you can try finding the element by its ID or XPath.
Finally, if all else fails, you can use WebDriverWait to wait for the element to be attached to the DOM before interacting with it.
Here is some code that illustrates how to handle a stale element exception:
try {
// find and interact with element
} catch (StaleElementException e) {
driver.navigate().
refresh();
// find and interact with element again
What is Stale Element Exception Why It Will Occur?
If you’ve ever encountered a “stale element reference exception”, it can be extremely frustrating. This error can occur for a number of reasons, but most often it happens when the page you’re trying to interact with has changed since the time your script loaded. In other words, the element you’re trying to find is no longer in the same place, or even on the same page.
There are a few different ways that this can happen:
-The element may have been deleted from the page entirely.
-The element may have been replaced with another element.
-The page may have been refreshed.
-You may have navigated to a different page and then back again.
In any of these cases, Selenium will throw a stale element reference exception because it is unable to locate the element on the page.
So how do you fix this? The best way is to simply find the element again using one of Selenium’s methods like findElement() or findElements(). By doing this, you’ll be able to refresh Selenium’s reference to the element and avoid getting a stale element reference exception.
How to Handle Stale Element Exception in C#?
When you are working with Selenium Webdriver, you will sometimes come across the “StaleElementReferenceException”. This exception occurs when the element you are trying to interact with is no longer attached to the DOM. This can happen for a number of reasons, but the most common one is that the page has been refreshed while the element was still in use.
There are a few ways to handle this exception. The first is to simply catch it and move on. This is not always possible, depending on what your test is trying to do.
If you are able to catch it and move on, then you can add a try/catch block around the code that is interacting with the element:
try {
//code that interacts with element
} catch (StaleElementReferenceException e) {
//move on or take some other action here
}
Another way to handle this exception is by using an Explicit Wait. With an Explicit Wait, you tell Selenium WebDriver to wait for a certain amount of time before timing out.
This gives the page enough time to load or refresh before continuing with your test. You can set up an Explicit Wait like this:
var wait = new WebDriverWait(driver, TimeSpan.FromSeconds(10));
IWebElement myDynamicElement = wait.Until
How to Handle Stale Element Exception in Selenium with Java
Conclusion
If you’re working with WebDriver and you’ve ever encountered a “stale element reference exception”, then this blog post is for you. A stale element is one that is no longer attached to the DOM, which can happen for a number of reasons. The most common reason is that the page has been refreshed or navigated away from before the element was located.
There are a few ways to handle this exception, but the most important thing to remember is that you need to find the element again. You can do this by using the driver’s findElement method, or by using one of the helper methods provided by WebDriver. Once you have found the element again, you can interact with it as usual.