How to Free an Array in C
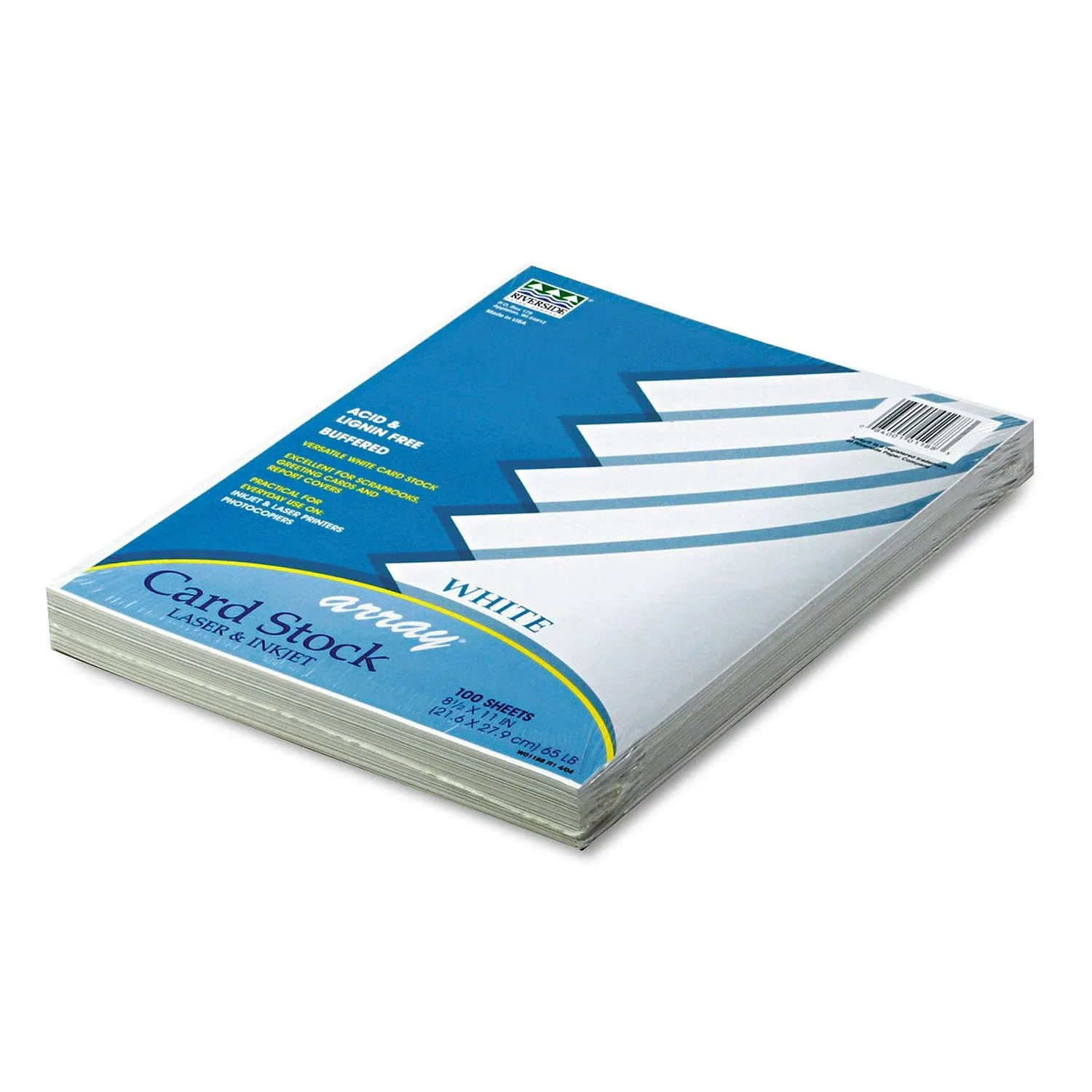
When you no longer need an array, you can free the memory associated with it by using the free() function. This function takes a single argument, which is a pointer to the array that you want to free. For example, if you have an array named myArray, you would free it like this:
- Allocate memory for the array using malloc or calloc
- Initialize each element in the array
- Use free to deallocate the memory when you no longer need it
How to Free an Array in C++
When working with arrays in C++, it is important to remember to free the array when you are finished with it. Failing to do so can lead to memory leaks. There are a few different ways to free an array in C++.
The most common way is to use the delete[] operator. This will delete all of the elements in the array and then free the array itself. For example:
int* myArray = new int[10]; // Use myArray… delete[] myArray;
Another way to free an array is by using std::vector::clear(). This will clear all of the elements from the vector, but it does not free the underlying array.
The vector class will take care of that for you when it goes out of scope or is destroyed. For example:
How to Free an Array of Pointers in C
When working with arrays of pointers in C, it is important to remember to free the memory associated with each pointer when you are finished using it. This can be done by looping through the array and calling the free() function on each pointer.
It is also important to set each pointer to NULL after freeing it, so that you do not accidentally try to use freed memory.
Failing to free memory or setting pointers to NULL can lead to memory leaks, which can eventually cause your program to crash.
Here is an example of how to free an array of pointers:
int i;
for (i=0; i < num_pointers; i++) {
How to Free an Int *Array in C
If you have an int array in C, you can free it using the free() function. This function will take a pointer to the array and will deallocate the memory that was allocated for it.
To use free(), you must include the stdlib.h header file.
Then, you can call free() like this:
free(array);
After you free the array, you should set the pointer to NULL so that it cannot be accidentally used again.
How to Free 2D Array in C
When allocating a 2D array, you need to use a loop to allocate each row of the array. The number of columns is fixed, but the number of rows can be variable. For example, if you have an array with 5 rows and 3 columns, you would use this code:
int **array; // Allocate the array
array = malloc(5 * sizeof(int *)); // Allocate each row
for (i=0; i<5; i++) {
array[i] = malloc(3 * sizeof(int)); }// Deallocate the array
How to Free an Array of Strings in C
When you need to free an array of strings in C, there are a few different ways you can do it. One way is to use the free() function for each string in the array. Another way is to use the calloc() or malloc() functions to allocate memory for the array, and then use the memset() function to set each element of the array to NULL.
If you’re using malloc(), you should first allocate enough memory for the number of strings in the array plus one more element for the terminating NULL character. Then, you can loop through the array and use strcpy() to copy each string into its allocated space. Finally, don’t forget to add a terminating NULL character at the end of your new array!
Here’s an example of how this might look:
#include
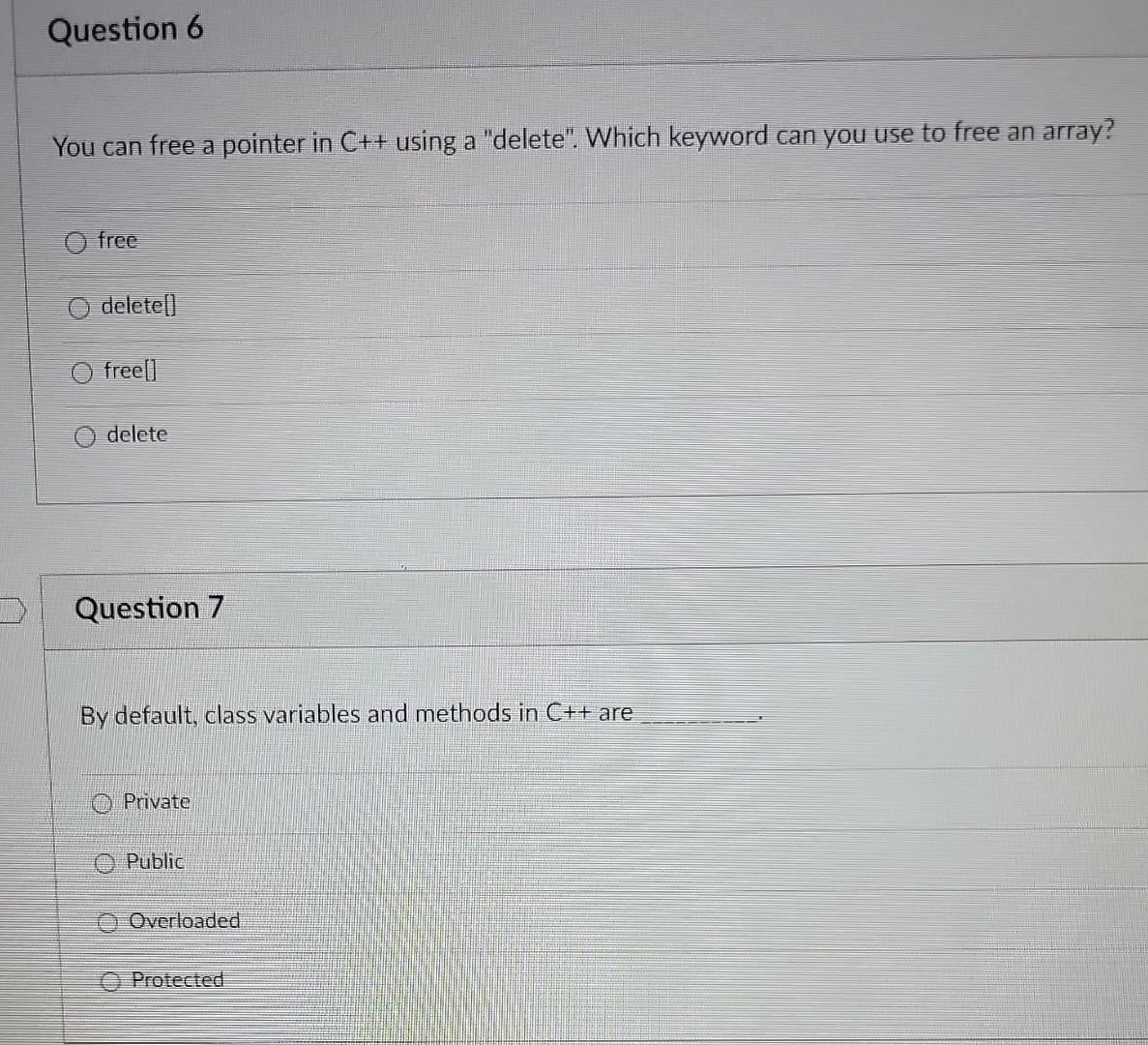
Credit: www.chegg.com
How to Free an Entire Array in C?
When you no longer need an array, you can free the memory associated with it by using the free() function. This function takes a single argument, which is a pointer to the array that you want to free. For example, if you have an integer array called my_array, you would free it like this:
int *my_array; /* Allocate and initialize my_array */ /* Use my_array */ free(my_array); /* my_array is now freed */
You can also use free() on arrays that have been allocated dynamically, using malloc(), calloc(), or realloc(). Simply pass in the pointer that was returned by one of those functions and the memory will be deallocated.
It’s important to note that once you’ve freed an array, its contents are no longer valid. You should not try to access any elements of the array after calling free(). Doing so could lead to undefined behavior in your program.
Can You Free Array in C?
Yes, you can free an array in C. To do so, you must use the free() function. This function takes a pointer to the array as its argument and frees the memory associated with that array.
How to Clear the Array in C?
Assuming you are using the C programming language, there are a couple different ways to clear an array. One way is to set all of the elements in the array to 0. This can be done by looping through each element in the array and setting it equal to 0.
Another way is to use the memset() function which takes 3 arguments-the address of the array, the value you want to set each element in the array equal to, and finally the number of bytes you want to set.
What Does Free () Do in C?
When you call free() in C, it deallocates memory that was previously allocated by a call to malloc(). This means that after calling free(), the memory is no longer reserved for your program and can be used by other parts of the system.
The pointer that you pass to free() must be a pointer that was previously returned by malloc(), calloc(), or realloc().
If you try to free a pointer that wasn’t allocated by one of those functions, you will probably get an error.
It’s important to note that free() only frees the memory itself, it doesn’t change the value of the pointer that you pass to it. So if you have a variable pointing to some allocated memory, after calling free() on that memory, the variable will still point to where the freed memory was located.
You’ll need to set the variable pointing to freed memory to NULL if you want to avoid any potential errors.
Releasing the Dynamically Allocated Memory using free()
Conclusion
In C, an array is a block of memory that is allocated at compile time. The size of the array is fixed and cannot be changed. To free an array, you must use the free() function.
This function will deallocate the memory associated with the array.